rolo
Member
Hello,
Supposse I have this CSV file:
ID,Name,Salary
1,Peter,12300
2,Bob,32000
3,Mary,37800
4,Mick,28900
5,Luke,15900
Then from Excel, I need some VBA code to fill "ID" in cell B1, change "Name" or "Salary" data and then save that record back to the CSV (in this example overwrite 3rd record of csv file).
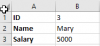
Is it possible to do it without opening the csv file
and only update the changed record, not the entire data set?
Thanks!
Supposse I have this CSV file:
ID,Name,Salary
1,Peter,12300
2,Bob,32000
3,Mary,37800
4,Mick,28900
5,Luke,15900
Then from Excel, I need some VBA code to fill "ID" in cell B1, change "Name" or "Salary" data and then save that record back to the CSV (in this example overwrite 3rd record of csv file).
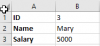
Is it possible to do it without opening the csv file
and only update the changed record, not the entire data set?
Thanks!